Top level expression is expected to have unit type
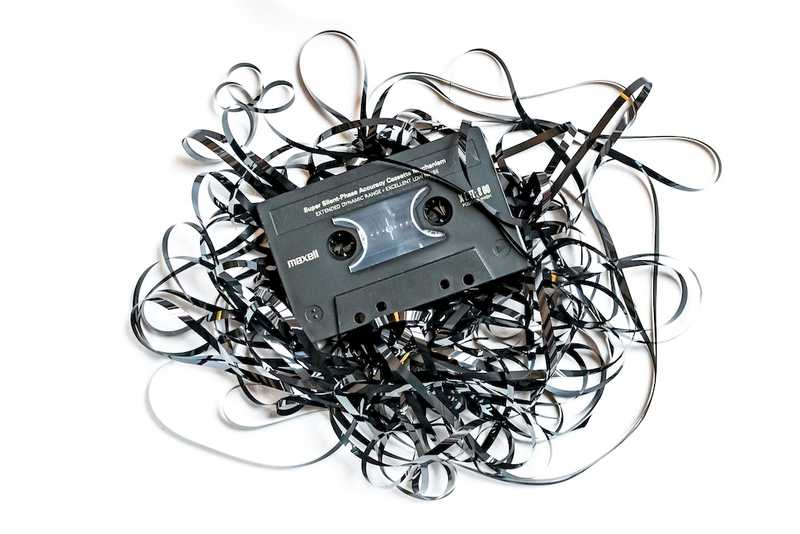
This error:
Top level expression is expected to have unit type
happens from time to time.
The error message is not so straigth forward and will probably be improved in near future. Let’s take a look at how to solve it and what it means
- Unit is Rescript’s equivalent of Javascript’s undefined.
- Top-level means that it’s defined within the module (or the file which is also a module). Within a function is not at top-level.
function getNumber () =>{
3 // this is fine, doesn not cause any errors because it's not at a top level
}
getNumber() //this is at top-level
or just
"hello there"
then you’ll then get an error if it’s at a top-level.
To fix the above examples there are two solutions. You can either do:
let _ = getNumber()
let _ = "hello there"
or
getNumber()->ignore
"hello there"->ignore
The only time where this is not an issue is when the type is actually unit type. This for example would not give us this error message.
()
let getNothing = () => {
()
}
getNothing
Another scenario when you could get this error is when you define a variable without assigning anything to it
let a
But if you do it inside a function you’ll have a different kind of error message
let testFunction = () => {
let x //error message: Did you forget a `=` here?
}
This is because at a top level Rescript always expects every expression to have type of unit to indicate that they don’t return a meaningful value. It’s a design choice that aligns with the language’s philosophy and purpose and there are many reasons for it that I won’t go into now. Most important is to be able to solve the error when it comes up :)
Hope this helped!